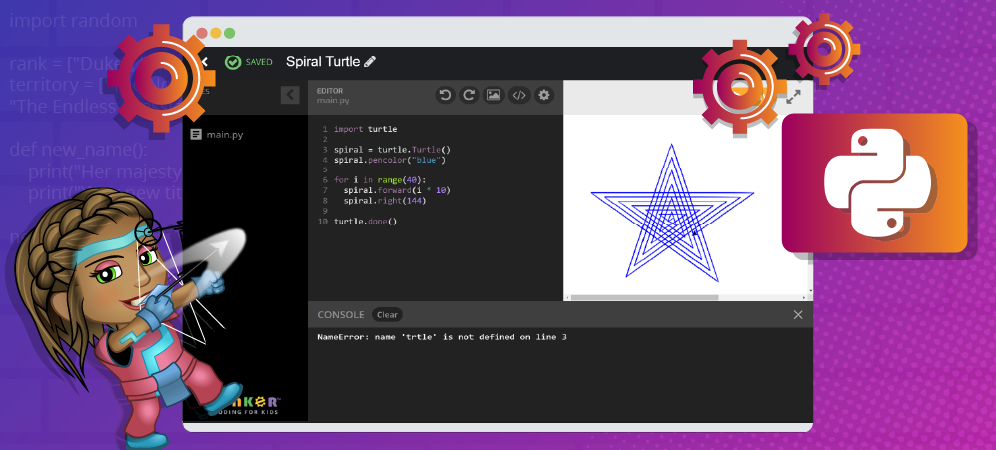
Did you know that you can code in your web browser using more than just Tynker Blocks?
Tynker supports Python, the text-based programming language used by millions of professional coders at places like Google, NASA, and IBM.
Python is a simple but powerful tool that anyone can learn, at any age, young or old. Python is a general purpose programming language: That means you’ll find Python used in a wide variety of professional fields like web development, statistics, scientific analysis, computer security, and more. Even journalists and business analysts use Python these days.
Python coding projects are growing in popularity as educational tools because it’s readable and high-level. Python has a welcoming and beginner-friendly community, as well as a flourishing ecosystem of powerful tools. Python has excellent libraries, tutorials, courses, books, and more, including some excellent online IDEs (integrated development environments), where you can experiment without installing anything. The language is named after the British comedy troupe, Monty Python.
Let’s see how you can become a Pythonista yourself, as simple as Tynker! You don’t need to install anything—just follow along online at tynker.com.
Table of Contents
Your First Python Project
Login at tynker.com. From your dashboard, select +Create Project, then choose Python Project. You’ll see the Python Editor appear. Let’s take a closer look.
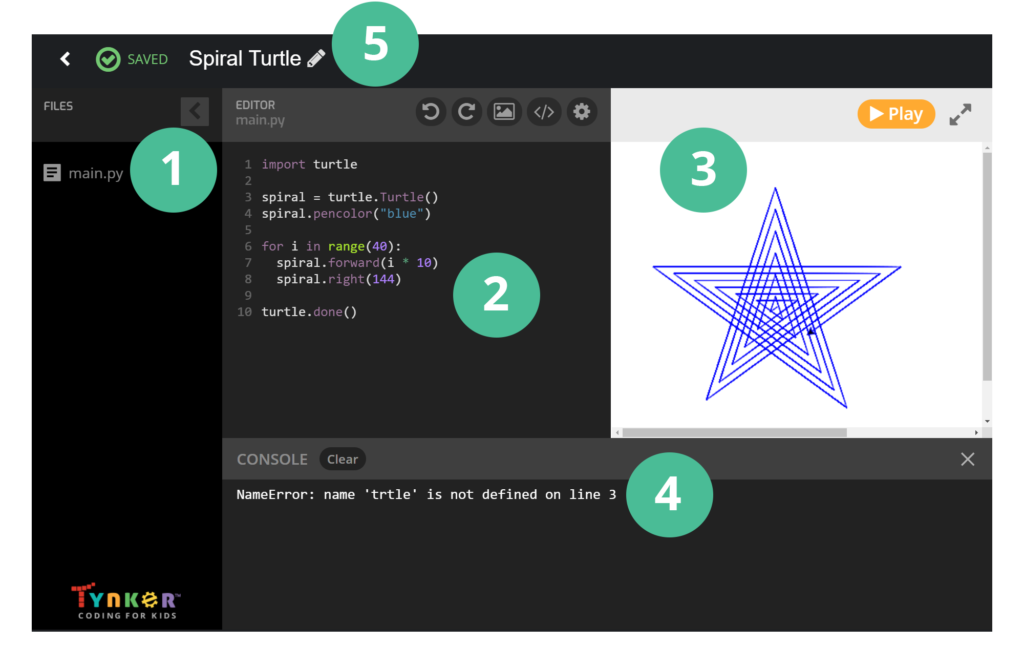
❶ This is the File Manager. Your Python programming project can be as simple as a single file, like this one named main.py. Advanced Python projects might contain several scripts, images, and other assets, organized into folders. Click on the ▾ next to the file names to Rename, Move, Download, or Delete a file.
❷ This is the Editor. Write your Python code here. Notice how line numbers in the Python code editor online appear on the left—this is a quick and easy way to refer to the particular parts of a Python program. (Don’t see anything to edit? First select a file from the File Manager.)
❸ This is the Output Area. You’ll see the results of what you created using our Python coding tool displayed here. Click Play to run your code.
❹ This is the Console. If you made an error in your code, you’ll get a notification here and some hints about what’s wrong, including the line number where the error appears. The Console pane won’t appear until you receive an error message (or use the console for another purpose). Click Clear to remove old messages, or the (X) to close the console entirely.
❺ Notice, too, that you can edit your project’s Title.
Code Snippets and Settings
The top of the code editor also has some special features worth pointing out.
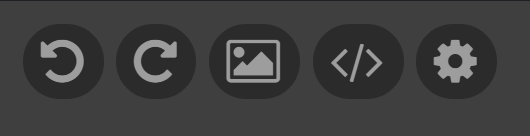
Undo. Revert an edit.
Redo. Undoes your last undo.
Media Library. Add images to your project. Then they’ll appear in the File Manager.
Code Snippets. Forget the syntax for a coding concept, like a for loop or how to get user input? Click this button to reveal some pre-written code that you can Insert directly into your project.
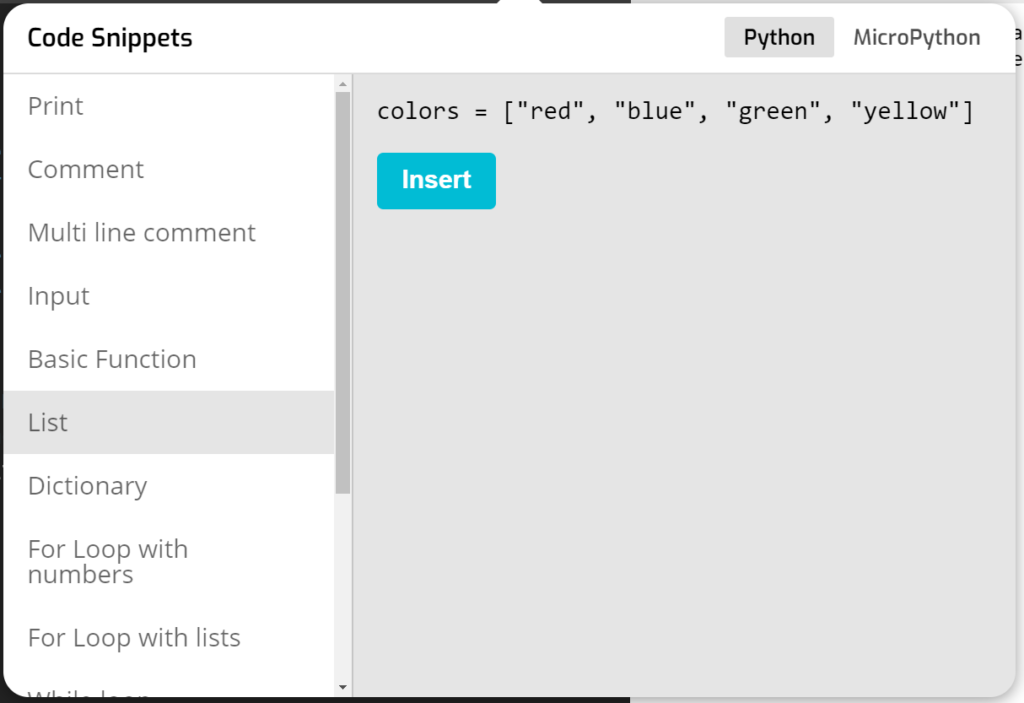
⚙️ Settings. Customize the editor’s display. For example, you can swap themes from Light to Dark.
Looking for some simple Python programs to see the possibilities? Read on.
Project #1: Fantasy Name Generator
How about a random name generator! This program generates goofy medieval titles. Just read the code closely to start.
nobles.py
import random
rank = ["Duke", "King", "Baron", "Earl", "Jester", "Queen", "Warden", "Knight", "Emperor"]
territory = ["The North", "The Basement", "Northumbria", "Dubuque, Iowa", "The Frozen Wastes", "The Endless Grasslands", "Tlon", "Mordor"]
def new_name():
print("Her majesty blesses you with a new position and rank.")
print("Your new title is " + random.choice(rank) + " of " + random.choice(territory))
new_name()
One list called rank holds all possible titles, while a second list called territory holds the possible lands you might rule over. The program randomly selects one element from rank and territory, then joins them together using the + operator.
Write a version of it yourself and press Play. Don’t like your luck? Re-run the program and get a new title. Here’s are a few sample runs, so you get the idea of what this program should output and how you might tweak it:
Her majesty blesses you with a new position and rank.
Your new title is Emperor of The Endless Grasslands
Her majesty blesses you with a new position and rank.
Your new title is King of Dubuque, Iowa
Her majesty blesses you with a new position and rank.
Your new title is Baron of The Basement
Change the name generator program so that it’s your favorite theme or fictional world. Can you add code so that this program gives you a henchman at random too? What about new honorifics or random adjectives? Perhaps you’re the Honorable Milkman or the Desperate Duchess.
HINT: Create another list variable (like rank and territory), then edit the new_name() function definition. Play around with it!
Project #2: How Much Spare Change
Here’s a Python script that defines a function called spare_change() — then gets the user’s input to calculate the worth of a handful of coins.
sparechange.py
def spare_change(quarters, dimes, nickels, pennies) :
cents = quarters * 25 + dimes * 10 + nickels * 5 + pennies
return cents
q = int(input("How many quarters? "))
d = int(input("How many dimes? "))
n = int(input("How many nickels? "))
p = int(input("How many pennies? "))
print("You have " + str(spare_change(q, d, n, p)) + " cents!")
Program it yourself, then press Play to see it in action. Here’s a sample run:
How many quarters? 5
How many dimes? 2
How many nickels? 4
How many pennies? 23
You have 188 cents!
Can you write your own program that asks the user a question, then makes a calculation based on their answer? Here are a few ideas:
- Ask the user how much they weigh, in pounds. Then reply with how much the user weighs in tangerines … paperclips … or elephants? Or how much they’ll weigh on the Moon!
- Calculate how many golf balls you’d need to stack to reach a distance, in miles. (Hint: A golf ball is 1.68 inches wide.)
- Calculate a duration: Convert a time given in weeks to a time in seconds.
Where to Go From Here?
Python coding is undeniably more challenging than coding with Tynker blocks—as you’ve noticed already, you have to spell everything exactly right and be careful about punctuation and spacing, too.
But Python is also rewarding: it unlocks powerful new tools and countless new avenues for the study of computer science.
If you’re hitting the limits of block coding, or considering your next steps in CS education, definitely check out Tynker’s Python courses, which are perfect for kids (and kids-at-heart, too) who want to learn something new about coding:
- Python 101 is an engaging interactive course suitable for readers of any age. Start by solving movement puzzles and brushing up on core coding concepts — before you know it, you’ll be designing games like Snake, Frogger, and Tetris.
- Python 201 is a text-driven course that takes a deeper dive into syntax, with interactive examples and exciting coding challenges.
- Data Science 1 is a crash course in basic statistics and data visualization techniques. Data is everywhere around us, transforming our world. Learn how to make use of Python, the most popular language for data science — and create beautiful data visualizations.
- Micro:Python is an advanced Python course for those interested in controlling gizmos and hardware gadgets with code. Connect a micro:bit to your computer and get coding!
Looking for something free? Find more starter projects in the Python category, too:
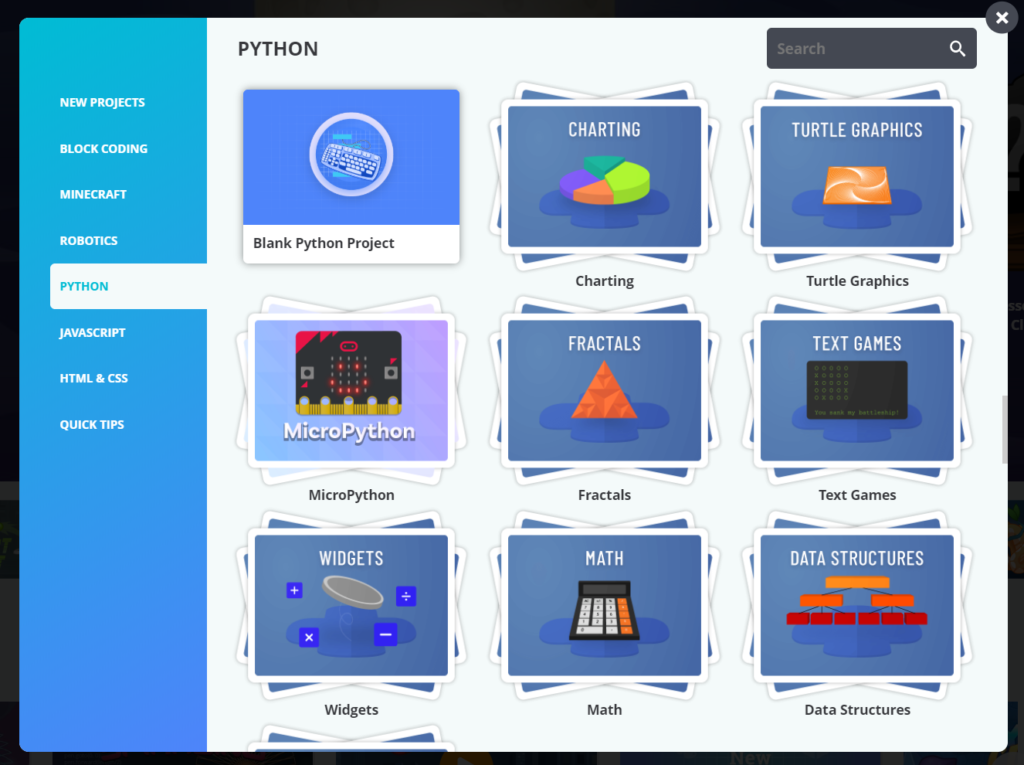
Did you make something cool with Python? Tell us at community@tynker.com.
More Activities
Looking for more coding activities? Check out the Course Catalog.
And read the rest of our free tutorials in the Tynker Toolbox series:
- Tynker Workshop Basics — Learn about coordinates and start coding with Tynker.
- The Animation Tool — Learn about frame-based animation and other animation tips.
- The Character Creator — Take control of custom rigs using the Animation blocks.
- Text Tricks — Work with speech bubbles and more. Tell your own stories, put on a play, or make a computer write poetry!
- The Sound Blocks — Play music with code! Add custom sound effects, too. Tynker’s brand new music tool supports MIDI and MP3.
- The Synth Blocks — Create your own sound effects and instruments! You can create crunchy dubstep drops, glitchy chiptunes, or instruments from any style of music you can imagine!
- Code Block Tricks — Get top-secret ninja tips for writing code fast in Tynker Workshop.
- The Pen Blocks — Make your actors draw as they move. Create patterns, draw geometric shapes, and more.
- The Physics Blocks — Create games or simulations with gravity, collisions, and more. Think: Angry Birds and Marble Madness.
- The Augmented Reality (AR) Blocks — Want to use video or photos in your programs? Try the AR Blocks, which let you code your own selfie!
- The Artificial Intelligence (AI) Blocks — Take your AR projects to the next level with face-, hand-, and pose-tracking. Explore what makes AI special.
- The Debugger — Learn about Tynker’s data debugger and get bug-fixing tips.
- The Tutorial Builder — Did you ever make a really cool Tynker Block project and wish you could teach the whole world exactly how you did it? Now you can!